Introduction
When I was trying to understand the insecurity of UNIX passwords, I looked for simple password cracking software as an example. Sure, there was the famous Crack, but I found Hale's viper.pl more readable. I wanted to improve the code to measure and print performance and time estimates for brute force attacks, so I contacted Hale about my ideas including re-writing his program in 'C' for maximum speed.
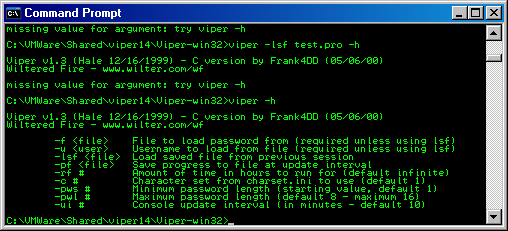
I wanted to know how long it takes to check for every possible password combination on different computing platforms, for different character sets and for different password lengths. The results were highly interesting! But first, we need to look into more details how passwords work and how they are generated. Concentrating on UNiX passwords, there is good reading material about them here. After reading it, writing a password cracker is not that hard. The basic design of a brute-force password cracker is a loop in which we continuously compute password hashes from incremented password strings of a generic character set - and compare the result to the real password hash until they match. Lets assume the password just contains lowercase letters from 'a' through 'z', we would start hashing and comparing:
'a', 'b', 'c', 'd'... 'w', 'x', 'y', 'z', then
'aa', 'ab', 'ac', 'ad' ... 'zw', 'zx', 'zy', 'zz', then
'aaa', 'aab', 'aac', 'aad' ... 'zzw', 'zzx', 'zzy', 'zzz'
... continuing to the maximum password length until we find the 'winning' combination. The faster it runs the better, because simple math tells us how many password string combinations we need to check. Using the example of a password with max. 8 characters (all lower case), we can calculate the total number of combinations (example for the 26 lowercase letters of the english alphabet):
(26)+ | 261+ |
(26x26)+ | 262+ |
(26x26x26)+ | 263+ |
(26x26x26x26)+ | 264+ |
(26x26x26x26x26)+ | 265+ |
(26x26x26x26x26x26)+ | 266+ |
(26x26x26x26x26x26x26)+ | 267+ |
(26x26x26x26x26x26x26x26) | 268 |
= 217,180,147,158 combinations! |
Considering that passwords not only have lowercase characters, but can be a mixture of lowercase, uppercase, numbers and special characters, the number of combinations to search increases drastically (see table).
Search spaces for other character sets:
character set 1 (62 characters): a-zA_Z0-9
character set 2 (93 characters): a-zA_Z0-9!@#$%^&*()_+-=[]{}\|;':",./<>?`
Password Length | Combinations for Character Set 1 | Combinations for Character Set 2 |
---|---|---|
1 | 62 | 93 |
2 | 3844 | 8649 |
3 | 238328 | 804357 |
4 | 14776336 | 74805201 |
5 | 916132832 | 6956883693 |
6 | 56800235584 | 646990183449 |
7 | 3521614606208 | 60170087060757 |
8 | 218340105584896 | 5595818096650401 |
total | 221919451578090 | 5656642206396600 |
In order to go through the vast search space as fast as possible, it is important to increase the hash computation speed. For the standard UNIX 'crypt' hash generation, the UFC-crypt package is optimized for different hardware with 32-bit and 64-bit CPU's. Adding a counter and a timer to the crack program will tell how many 'crypts' (and comparisons) can be done per second, giving us a estimate of the total time needed to search through all combinations of a character set. Compiling the program on different hardware and operating systems will give us an idea how systems compare in their speed for cracking passwords. The first results on a 650 Mhz Pentium III system showed consistent 50,000 c/s (cracks per second). Let's calculate the time for all combinations:
total # combinations / 50,000 cracks/sec = total number of seconds needed
5656642206396600 / 50,000 cracks/sec = 113132844128 secs, / 86,400 (secs per day) = 1309408 days, / 365 (days per year) = 3587 years !!!
It will take about 3587 years to go through all possible combinations of a 93-char long character set for a 8-char password.
But what if the number of combinations is reduced, say by shorter passwords, by not using special characters, numbers or mixing upper/lowercase characters? Here are the estimates using the same character sets from page 2, with a speed value of 50,000 cracks/s:
Password Length | time needed for Character Set 1 | time needed for Character Set 2 |
---|---|---|
1 | < 1 sec | < 1 sec |
2 | < 1 sec | < 1 sec |
3 | 4.8 secs | 16 secs |
4 | 5 mins | 25 mins |
5 | 5.17 hours | 1.63 days |
6 | 13.4 days | 151.4 days |
7 | 2.27 years | 38.6 years |
8 | 140.7 years | 3587.4 years |
Now its easily understandable why password standards are raised and enforced as any weakness makes brute force attacks more and more likely to be successful.
Conclusion: Going for a 8-char Unix password brute force on a PC is still tough. :) But 5- or 6-char Unix passwords are a piece of cake for anybody and should not be used! Numbers and special characters should be utilized in passwords to vastly increase the search space. Passwords should not be words found in dictionaries, not even with slight alterations. Alternate, slower computing password encryption schemes (i.e. MD5) should be used. Or, maybe, passwords should be abandoned at all, in favor of safer technologies. Recent development of "Rainbow Tables" allow to circumvent the password computing and can search through huge, pre-sorted password hash files at I/O speeds.
Thanks
Thanks to Hale www.deviance.org as the original author of viper.pl, to the authors of UFC-crypt at the Free Software Foundation, to the authors of OpenSSL, and David C. Rankin for bugfixes.
License
Viper is freeware, provided the original author and source information remains. [Download Here]
Sample Run
susie112:/home/me/viper-1.6/src # ./viper -f passwd -u root -ui 1 -v Viper v1.6 (Hale 05/12/2000) - C version by Frank4DD (05/05/2014) Wiltered Fire - www.wilter.com/wf, incl. bugfixes by David C. Rankin Found: user root pw:reUJbHrFWYCQk Found: Charset 0 in charset.ini ...command line parameters loaded. Character set is 93 chars long Starting crack on: Sun Oct 3 23:04:44 2009 Cracking for pass length 1 (93 possibilities) Cracking for pass length 2 (8649 possibilities) Cracking for pass length 3 (804357 possibilities) Cracking for pass length 4 (7.48052e+07 possibilities) [ Length: | Last: | CPS: | Time Spent: | Time Remaining: | Done: ] ------------------------------------------------------------------------------- [ 4 | kq2r | 150000 | 000d:00h:01m:00s | 000d:00h:07m:18s | 12.03% ] The password has been located. Username : root Password : test Started : Sun Oct 3 23:04:44 2009 Finished : Sun Oct 3 23:06:30 2009 Duration : 000d:00h:01m:00s Viper exiting...
Speed Comparisons
What impact has different hardware and how fast are different systems? How does increasing processor power improve the brute-force cracking speed? The table below has some numbers:
System | CPU | OS | compiler | speed in c/s |
---|---|---|---|---|
Noname Desktop | 1x 650 Mhz Pentium III | Windows 98 | gcc | 51,282 |
same | same | Linux 2.2.13 | gcc | 39,062 |
SUN Server E-250 | 2x 400 MHz UltraSparc | Solaris 2.6 | gcc | 24,691 |
HP WS Model 778 | 1x 180 Mhz PA-Risc | HP-UX 10.20 | gcc | 6,993 |
HP Laptop | 1x 1.7 GHz AMD 64 | Windows XP | gcc | 121,212 |
Virtual Server | 1x CPU, shared | Linux 2.6.31 | gcc | 150,000 |